Next.js Explained: What It is Used For, Frontend or Backend, and How It Compares to React And Angular
Explore how Next.js is used to build full-stack web applications using the new App Router, and learn whether it’s better than plain React or Angular.
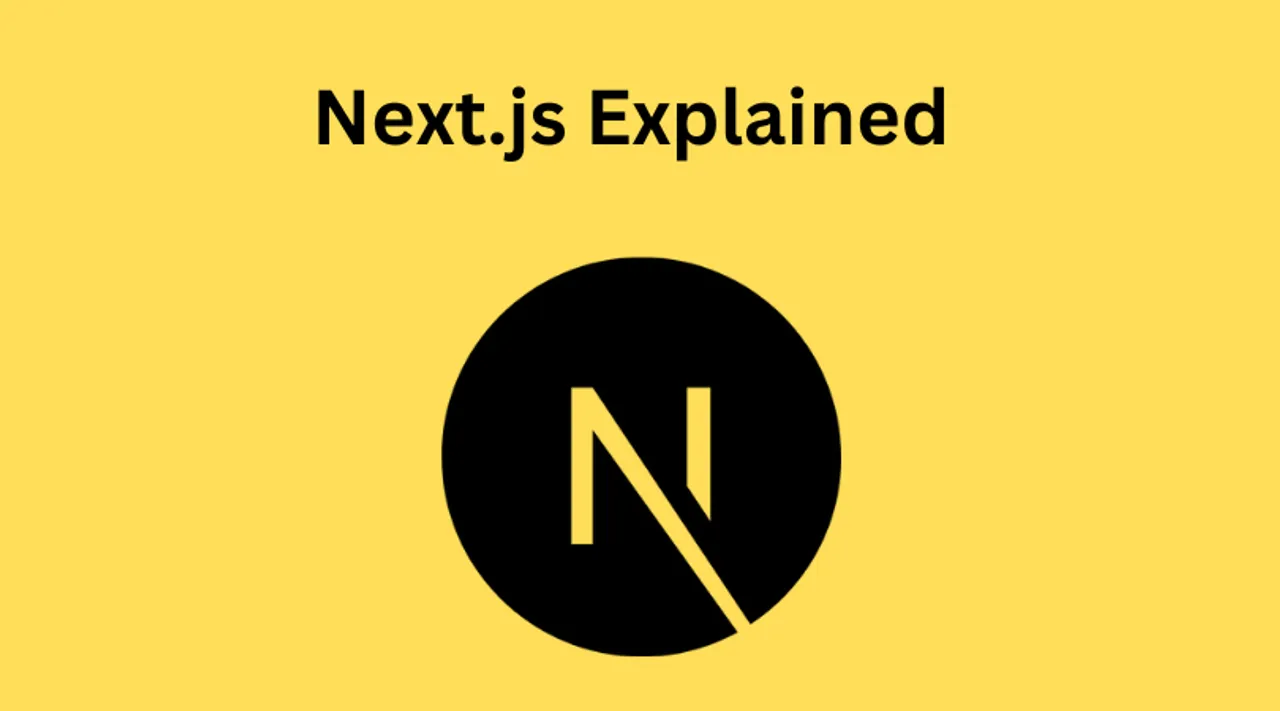
Next.js has evolved significantly over the years—now offering powerful features via its new App Router. Whether you’re building SEO-friendly content sites, high-performance dashboards, or dynamic full-stack applications, Next.js streamlines your development process by integrating both frontend and backend capabilities into one cohesive framework.
In this post, we’ll answer some common questions:
- What is Next.js used for?
- Is Next.js better than React?
- Is Next.js frontend or backend?
- Is Next.js better than Angular?
Let’s dive into these questions and see how the new App Router is reshaping the Next.js experience.
What Is Next.js Used For?
Next.js is a React framework designed to simplify the process of building production-grade web applications. With Next.js you can:
- Build SEO-Friendly Sites: Server-side rendering (SSR) and static site generation (SSG) ensure your pages are pre-rendered for search engines.
- Leverage the New App Router: In Next.js 13+, the App Router (via the
app/
directory) introduces:- Nested layouts and shared UI: Create reusable layouts that persist between navigations.
- React Server Components and Streaming: Fetch data directly in your components with async/await and stream content progressively.
- Incremental Adoption: You can migrate one route at a time while still using the Pages Router.
- Develop Full-Stack Applications: With built-in API routes (or Route Handlers in the App Router), Next.js lets you handle backend logic seamlessly alongside your frontend.
Code Example: A Simple App Router Page
Below is a quick example that shows a Next.js page in the new app
directory:
// app/page.tsx
export default async function HomePage() {
// Data is fetched server-side automatically
const res = await fetch("https://jsonplaceholder.typicode.com/posts", {
cache: "force-cache",
});
const posts = await res.json();
return (
<div>
<h1>Welcome to Next.js!</h1>
<ul>
{posts.slice(0, 5).map((post) => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
This snippet demonstrates how the new App Router allows you to directly fetch data in an async component that renders on the server.
Is Next.js Better Than React?
It is very important to note that Next.js is built on React. In other words, it does not replace React, it rather enhances it by providing additional features:
- Routing and File-Based Navigation: Instead of manually setting up routing with libraries like React Router, Next.js automatically maps files (or folders, with the App Router) to routes.
- Server-Side Rendering & Static Generation: Next.js offers SSR, SSG, and Incremental Static Regeneration (ISR) out of the box.
- Improved Data Fetching: With the new App Router, data fetching becomes simpler and more intuitive through async server components.
If you are already comfortable with React, Next.js gives you the tools to build better-performing, production-ready applications with less boilerplate.
Is Next.js Frontend or Backend?
Next.js is a full-stack framework. While its primary strength is in building rich, interactive frontend UIs with React, it also provides robust backend features:
- Frontend: Uses React to build the user interface. With the App Router, you can now create complex, nested layouts and leverage React Server Components.
- Backend: Next.js includes API routes (or Route Handlers in the App Router) that allow you to create server-side endpoints directly within your project. This means you can handle tasks like authentication, form submissions, and data processing without needing a separate backend.
Thus, Next.js allows you to develop a complete application—both client and server—using one unified codebase.
Is Next.js Better Than Angular?
Comparing Next.js and Angular involves looking at different philosophies and use cases:
- Next.js (with React):
- Flexibility: Leverages the simplicity and modularity of React along with powerful SSR/SSG features.
- Incremental Adoption: You can start small with Pages Router and gradually move to the App Router as your application grows.
- Developer Experience: Easier to learn if you’re already familiar with React. Great tooling, automatic code splitting, and a thriving ecosystem.
- Angular:
- Full-Fledged Framework: Offers a comprehensive solution with built-in state management, dependency injection, and a more opinionated architecture.
- Steep Learning Curve: Requires familiarity with TypeScript, RxJS, and Angular’s conventions.
- Enterprise Ready: Well-suited for large-scale, enterprise applications with strict structure.
While Angular is a powerful, all-in-one solution, many developers prefer Next.js for its flexibility, ease of use, and the ability to incrementally build and scale projects without being locked into one strict paradigm.
Who Uses Next.js ?
Next.js has become a preferred framework for numerous high-profile companies due to its performance, scalability, and developer-friendly features. Notable adopters include Netflix, Twitch, Uber, Nike, WhatsApp, Starbucks, Hulu, Walmart, Apple, TikTok, Lyft, and Spotify. citeturn0search0turn0search24
Hosting A Next.js App
Hosting a Next.js project is straightforward with Vercel which is developed by the creators of Next.js. Vercel is a serverless platform that integrates effortlessly with headless content, commerce, or databases, making it a top choice for deploying Next.js applications.
To deploy your Next.js project on Vercel,
-
Create a Vercel Account: Sign up on Vercel’s website, opting to continue with GitHub for a streamlined process.
-
Import Your Repository: After signing up, import your Next.js repository to Vercel. Grant vercel access to your repositories. Vercel will detect your Next.js app and apply optimal build settings automatically.
-
Deploy: Initiate the deployment, and within minutes, your Next.js application will be live, accessible via the provided URLs.
Alternatively, Next.js projects can be hosted on other platforms like Netlify, AWS,Cloudflare or through traditional server setups. However, Vercel’s tight integration with Next.js offers a particularly efficient and developer-friendly experience.
Conclusion
Next.js provides a modern, full-stack framework that leverages the strengths of React—while adding its own robust features like SSR, SSG, and the revolutionary App Router. Here’s a quick recap:
- Usage: Next.js is used to build SEO-friendly, full-stack web applications with advanced data fetching and routing capabilities.
- Built on React: It enhances React by handling routing, server-side rendering, and more.
- Full-Stack Capabilities: Next.js seamlessly integrates both frontend and backend logic.
- Comparison with Angular: While Angular is a complete, opinionated framework, Next.js offers flexibility and a gentler learning curve for React developers.
Related Posts
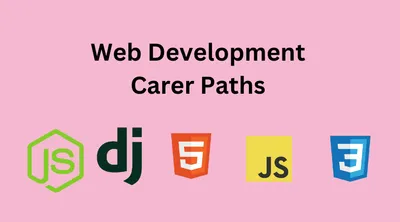
Navigating Your Web Development Career Path: A Roadmap for Success
Explore different career paths in web development, from frontend and backend to full-stack, and learn how to build a successful career in tech.
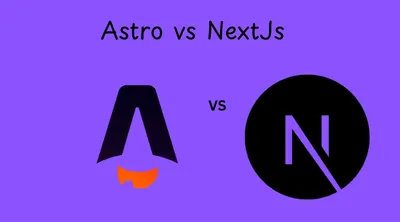
Astro JS vs Next.js: Choosing the Right Framework for Your Project
A comprehensive comparison between Astro JS and Next.js to help you determine the best framework for your web development needs.
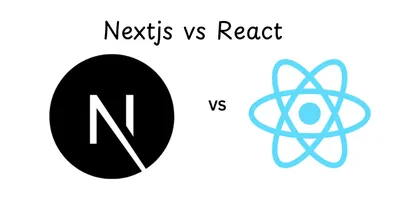
Next.js vs React: Choosing the Right Tool for Your Web Development Project
An in-depth comparison between Next.js and React to help you determine the best choice for your web development needs.